Amazon is an extensive platform with product listings divided across numerous pages. For efficient data collection, it is essential to navigate Amazon’s complex web structure and efficiently handle pagination.
This guide discusses various techniques to handle pagination in web scraping Amazon and some common errors that may occur.
Understanding Amazon’s Pagination Structure
Amazon’s pagination works using page numbers or token-based navigation. Most search results follow a URL pattern as shown:
https://www.amazon.com/s?k=laptop&page=2
Generally, the page parameter increments for each subsequent page. However, some product categories or filtered searches require additional handling and may use AJAX-based pagination.
Methods to Handle Pagination in Large-Scale Scraping of Amazo
1. Using URL Parameters to Iterate Pages
To scrape multiple pages from Amazon, you can automate the process by utilizing URL parameters. This method involves modifying the page parameter in the URL to access subsequent pages of search results.
This method of handling pagination by manipulating URL parameters is beneficial for structured data extraction.
It’s a fundamental technique that you can use if you want to collect large amounts of data from any e-commerce site.
Here’s how you can implement the method using the Python libraries Requests and BeautifulSoup:
-
Setting Up the Environment
Install all the necessary Python libraries. Requests handle the HTTP requests to Amazon, while BeautifulSoup parses the HTML content.
pip install requests beautifulsoup4
-
Defining the Base URL and Headers
First, you need to set a base URL for your search query on Amazon. To access different pages, you need to modify this URL dynamically.
Also, set up headers to include a user-agent for mimicking a real browser and prevent being blocked by Amazon’s anti-scraping measures.
BASE_URL = "https://www.amazon.com/s?k=laptop&page="
HEADERS = {"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64)"}
-
Iterating Through Pages
Use a loop to iterate through the number of pages you want to scrape. In the example given, the loop runs from 1 to 5, scraping the first five pages of search results for laptops.
for page in range(1, 6): # Adjust the range as needed for more pages
url = BASE_URL + str(page)
response = requests.get(url, headers=HEADERS)
soup = BeautifulSoup(response.text, "html.parser")
-
Extracting Data
Within each page, you can extract specific data, such as product titles. You need to identify class tags for product listings that Amazon typically uses by inspecting the page elements.
Here, span tags with certain classes are used to find product titles.
products = soup.find_all("span", class_="a-size-medium a-color-base a-text-normal")
for product in products:
print(product.text)
2. Extracting ‘Next Page’ Links Dynamically
Modifying URL parameters for pagination sometimes become obsolete, especially for website layout changes.
So, to maintain effective scraping, you need to adapt to changes in a website’s structure.
A dynamic link extraction technique can ensure that your scraper remains functional even if Amazon updates its pagination mechanism.
You can dynamically extract the URL of the Next Page button directly from the webpage.
Also, by automating the process of following pagination links, you minimize the risk of missing data.
This method is beneficial for large-scale scraping projects where maintaining data accuracy and completeness is essential.
-
Understanding Dynamic Link Extraction
In this method, you find the link element that Amazon uses to navigate to the next page of products.
Note that this element is part of the site’s live HTML. So extracting it dynamically allows your scraping process to adapt to changes in page layout without manual updates.
-
Implementing the Extraction with BeautifulSoup
Using BeautifulSoup, you can pinpoint the Next Page button by identifying its class name.
Generally, it is labeled something like s-pagination-next, which Amazon uses to denote pagination controls.
from bs4 import BeautifulSoup
import requests
# Example URL (starting page)
url = "https://www.amazon.com/s?k=laptop"
headers = {"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64)"}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
# Finding the 'Next Page' link
next_page = soup.find("a", class_="s-pagination-next")
if next_page:
next_page_url = "https://www.amazon.com" + next_page["href"]
print("Next page URL:", next_page_url)
else:
print("No more pages to scrape.")
-
Handling Multiple Pages
You can use a loop to follow the Next Page link to scrape across multiple pages continuously.
The process requests the new URL retrieved from the Next Page button, parses the returned HTML, and repeats the extraction of the next page link.
while next_page:
response = requests.get(next_page_url, headers=headers)
soup = BeautifulSoup(response.text, 'html.parser')
products = soup.find_all("span", class_="a-size-medium a-color-base a-text-normal")
for product in products:
print(product.text)
next_page = soup.find("a", class_="s-pagination-next")
if next_page:
next_page_url = "https://www.amazon.com" + next_page["href"]
else:
print("Finished scraping all pages.")
3. Using Selenium for JavaScript-Rendered Pages
Amazon pages load dynamically with JavaScript. So, sometimes, traditional HTTP request methods don’t work, and you need to use tools like Selenium to automate web browsers.
Selenium can interact with web elements, even those loaded by JavaScript, making it ideal for efficiently handling pagination like AJAX or infinite scrolling.
-
Setting Up Selenium
Set up Selenium with the appropriate web driver.
pip install selenium
Configure the driver to run in headless mode and run scripts on servers or environments without a GUI.
from selenium import webdriver
from selenium.webdriver.common.by import By
options = webdriver.ChromeOptions()
options.add_argument("--headless") # Enables headless mode
driver = webdriver.Chrome(options=options)
-
Navigating Pages
Navigate to the initial search page on Amazon. Here, you will begin the process of interacting with the page elements:
driver.get("https://www.amazon.com/s?k=laptop")
-
Extracting Data and Handling Pagination
You can locate and extract data such as product names or any other information rendered dynamically using Selenium.
Identify the correct CSS selectors that point to these data elements and proceed to handle pagination:
for _ in range(5): # Limit to first 5 pages for example
products = driver.find_elements(By.CSS_SELECTOR, "span.a-size-medium")
for product in products:
print(product.text)
# Attempt to click the 'Next Page' button
next_button = driver.find_element(By.CLASS_NAME, "s-pagination-next")
if next_button:
next_button.click()
else:
print("No more pages available or end of list reached.")
Break
-
Closing the Driver
Close the Selenium driver to free up resources and avoid leaving the browser running in the background:
driver.quit()
Common Errors and How to Fix Them
When scraping Amazon, you might face several common challenges. Understanding these challenges and knowing how to address them is crucial for effective scraping.
1. Getting Blocked (403 Forbidden or CAPTCHA)
Amazon’s robust anti-scraping technologies detect frequent scraping activities, which can lead to IP blocks or CAPTCHA challenges, which manifest as 403 Forbidden errors or CAPTCHA prompts.
To overcome such blocks, it is necessary to implement proxy use, mask the IP address, and distribute requests over a larger network.
Rotating user-agent headers in each request can also help simulate access from different browsers, further disguising scraping activities.
To mimic more natural browsing behavior, it is essential to introduce delays between requests.
import time
import random
import requests
# Example of implementing delays and rotating headers
headers_list = [
{"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64)"},
{"User-Agent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7)"}
]
for url in urls:
headers = random.choice(headers_list)
response = requests.get(url, headers=headers)
time.sleep(random.uniform(1, 3)) # Random delay between requests
Go the hassle-free route with ScrapeHero
Why worry about expensive infrastructure, resource allocation and complex websites when ScrapeHero can scrape for you at a fraction of the cost?
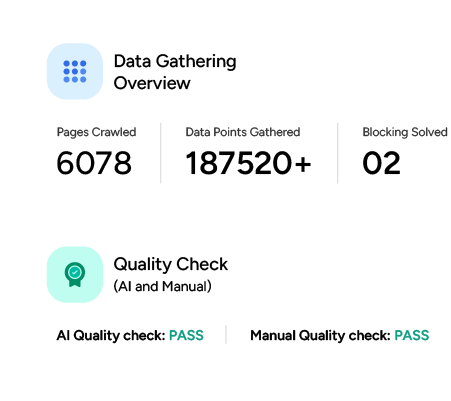
2. Empty or Partial Data
Empty or partial data issues can arise when the webpage content is loaded dynamically via JavaScript. The data becomes invisible to tools that only parse static HTML content.
To address this issue, you can use Selenium, which acts as a real browser, interprets JavaScript, and ensures content is captured.
Selenium automates browser interactions and retrieves the full content, including those revealed upon interacting with the page elements.
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://www.amazon.com/s?k=laptop")
products = driver.find_elements_by_css_selector("span.a-size-medium")
for product in products:
print(product.text)
driver.quit()
3. Broken Pagination
When a website’s layout changes, fixed pagination links may become useless if URLs that previously controlled page numbers no longer work.
To prevent this, you need to dynamically extract the links to the following pages directly from the page’s content.
Your script can automatically adjust to changes in the site’s pagination by using BeautifulSoup to find and follow the Next Page link on each page.
from bs4 import BeautifulSoup
import requests
url = "https://www.amazon.com/s?k=laptop"
while url:
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
next_page = soup.find("a", class_="s-pagination-next")
if next_page:
url = "https://www.amazon.com" + next_page['href']
else:
url = None
How ScrapeHero Web Scraping Service Can Help
To handle pagination in web scraping, you need to employ sophisticated technologies such as Selenium or IP rotation.
If you need extensive market analysis, competitor monitoring, or price tracking, you need uninterrupted data collection that can seamlessly navigate and manage pagination.
ScrapeHero web scraping service ensures a comprehensive solution for enterprise-scale data extraction projects.
We can tailor each website’s specific layout and dynamics by creating custom crawlers that traverse various pagination styles, from button-driven pages to infinite scrolls and AJAX-loaded content.
As a reliable and scalable partner, ScrapeHero ensures seamless data collection to meet the complex needs of modern enterprises.
Frequently Asked Questions
Pagination in scraping is the process of navigating through multiple pages of a website to gather complete data.
Handling pagination in large-scale scraping is crucial to ensuring a comprehensive dataset and avoiding missing essential data scattered across multiple pages.