Due to the growing prevalence of mobile devices, web scraping Android apps rather than desktop apps can offer many unique insights.
Mobile apps have higher engagement rates than desktop applications, and they provide location-specific data and mobile-first user experiences.
In this article, we discuss how to scrape data from the Amazon Android app using Python and some challenges you might encounter when web scraping an android app.
Step-by-Step Python Code for Scraping Data From Amazon App
1. Import Libraries and Establish Connection
a. You need to import the Device class from the uiautomator library to interact with the Android UI.
from uiautomator import Device
b. Now, you must initialize a connection to the Android device that’s connected for debugging.
# Connect to the default device
d = Device()
2. Launch the Amazon App
a. You can now turn on the device screen and navigate to the home screen.
d.screen.on()
d.press.home()
b. Click the Amazon app icon.
d(text="Amazon").click() # Assumes 'Amazon' is visible on the home screen
3.Navigate to Search and Perform a Search
a. Check for a search bar or icon and click it to focus on the search input.
if d(description="Search").exists:
d(description="Search").click()
b. You now have to set the text in the search input field to laptops and submit the search by pressing the enter key.
d(className="android.widget.EditText").set_text("laptops")
d.press.enter()
4.Extract and Print Product Titles
a. Delay execution for some time and wait for the search results to populate.
import time
time.sleep(5) # Allows time for search results to load
b. Capture the text elements from the search results and print each item’s title, including product names, descriptions, or prices.
# Extract and print product titles, including scrolling
while True:
products = d(className="android.widget.TextView")
for i in range(products.count):
product = products[i]
title = product.info.get('text', None)
if title:
print(title)
# Check if we can scroll down; if not, break the loop
if not d(scrollable=True).scroll.vert.forward():
print("No more products to display.")
break
5.Conclude the Script
You can now return to the home screen, ending the session.
d.press.home()
Note: You have to adjust for changes in layout and design when scraping the Amazon app.
Complete Code
from uiautomator import Device
import time
# Connect to the default device
d = Device()
# Ensure the device screen is on and go to the home screen
d.screen.on()
d.press.home()
# Launch the Amazon app by clicking on its icon
d(text="Amazon").click() # Assumes 'Amazon' is visible on the home screen
# Wait for the app to open and stabilize
time.sleep(2)
# Navigate to the search bar and click it
if d(description="Search").exists:
d(description="Search").click()
else:
print("Search bar not found, check the description used to locate it.")
# Enter the search term and execute the search
d(className="android.widget.EditText").set_text("laptops")
d.press.enter()
# Allow time for search results to load
time.sleep(5)
# Extract and print product titles, including scrolling
while True:
products = d(className="android.widget.TextView")
for i in range(products.count):
product = products[i]
title = product.info.get('text', None)
if title:
print(title)
# Check if we can scroll down; if not, break the loop
if not d(scrollable=True).scroll.vert.forward():
print("No more products to display.")
break
# Exit the app by pressing the home button
d.press.home()
What Are the Challenges of Web Scraping From Android Apps?
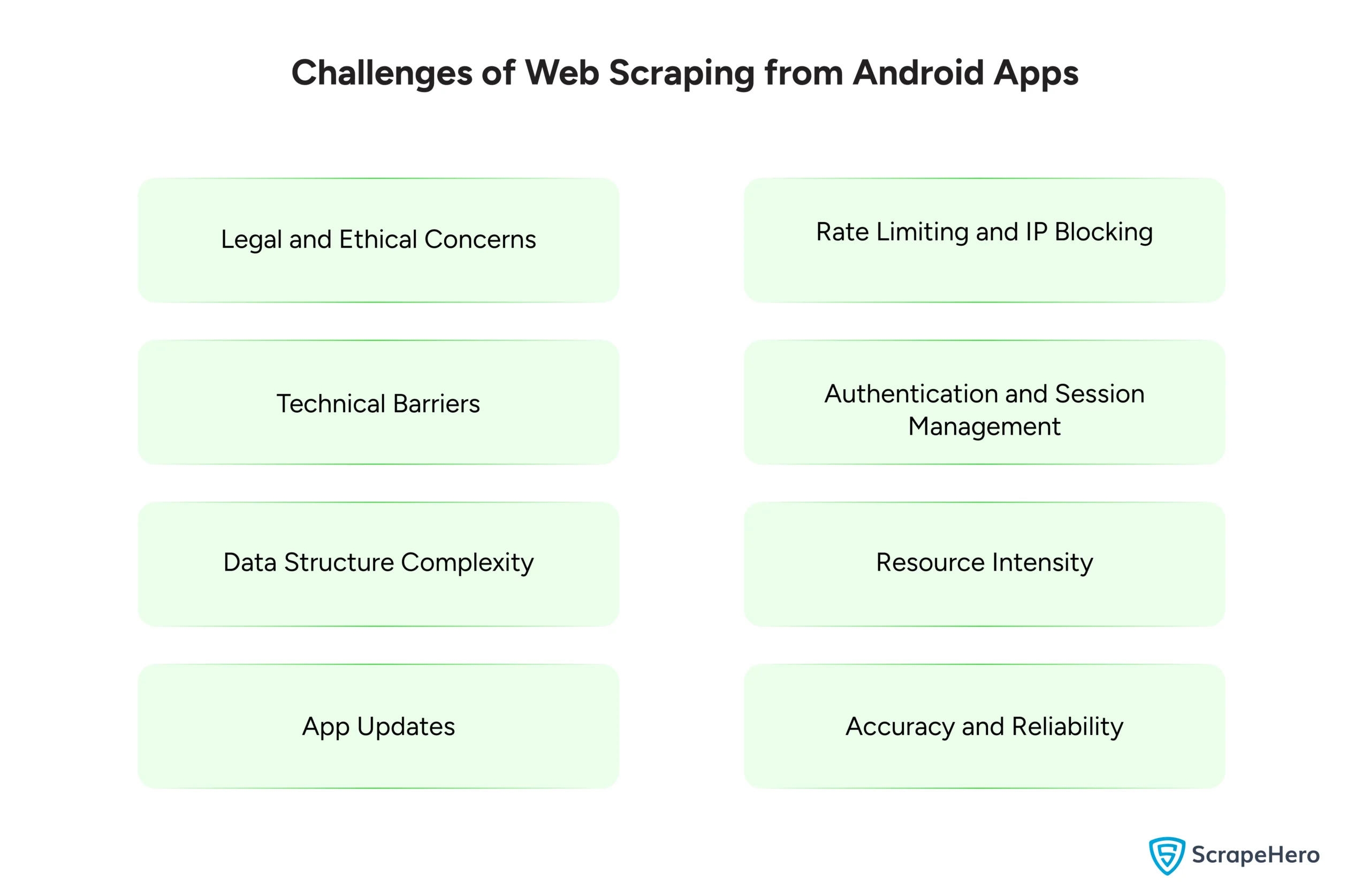
When web scraping Android apps, you will encounter several challenges that can complicate the process. Some of these challenges include:
1. Legal and Ethical Concerns
Web scraping raises ethical and legal concerns, as many Android apps prohibit scraping. If the data scraped includes personal information, then it can violate privacy laws like the CCPA.
2. Technical Barriers
Android apps use techniques such as CAPTCHA or encrypted data transmissions to prevent scraping and protect the app’s data.
You may have to bypass CAPTCHAs while web scraping to obtain the required data.
3. Data Structure Complexity
The structure of data within apps is complex, and it is nested within multiple layers of the app’s user interface.
Extracting this data requires advanced knowledge of data parsing, the tools, and the scripts used.
4. App Updates
When mobile apps are frequently updated, their structure, UI, or underlying APIs change, breaking the existing scraping scripts.
5. Rate Limiting and IP Blocking
Many apps implement rate limiting or IP blocking, which restricts data extraction within a specific time frame.
IP blocking from websites can only be prevented by techniques like using proxies and rotating IP addresses.
6. Authentication and Session Management
Some apps require login or authentication steps to access data. So, managing these sessions and authentication can be challenging for solid security measures.
7. Resource Intensity
Processing large amounts of data can be resource-intensive both in terms of computation and bandwidth.
8. Accuracy and Reliability
Due to several factors, such as errors in the script, the scraped data may be incomplete or incorrectly scraped, which can challenge its accuracy and reliability.
Wrapping Up
Data scraping from android apps provides businesses with strategy. However, web scraping android apps can also involve several challenges that need to be adequately addressed.
Writing scripts and maintaining scrapers by yourself is challenging as setting up a team involves extra time and cost.
So, enterprises need a reliable data scraping partner like ScrapeHero with technical expertise, especially for large-scale web scraping, to handle the challenges.
ScrapeHero’s web scraping service is capable of fulfilling all your data needs, which allows you to concentrate on your business rather than worry about technical details.
Frequently Asked Questions
Data scraping from Android apps involves using tools like uiautomator. These tools automate interactions with the app and extract data from its interface.
Android data scraping provides real-time data access and automation capabilities. Businesses can use the data to gain insights into user behavior and app performance.
We can help with your data or automation needs
Turn the Internet into meaningful, structured and usable data